Inter-Integrated Circuit
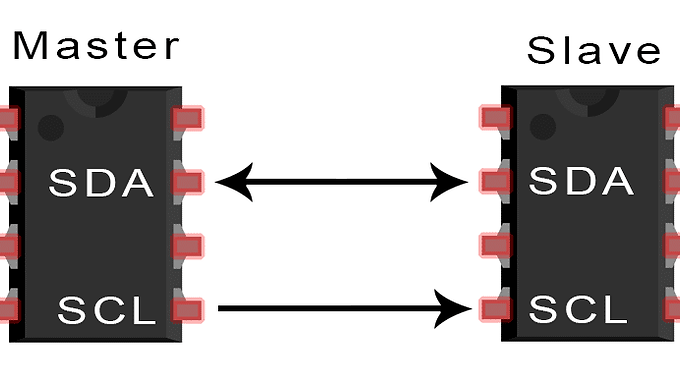
I2C stands for Inter-Integrated Circuit which is a widely used communication protocol in any imaginable electronic device with a microcontroller or a microprocessor . It is a fairly simple protocol as compared to Serial Peripheral Interface (SPI) Protocol.
I2C was introduced by NXP Semiconductors in the 1980s as a way to communicate between different parts of a TV, which has then been widely adapted over as a standard protocol due to its ease of access in embedded systems.
Features of I2C
I2C uses clock signals to synchronize the communication between two devices over a bidirectional data line. This is achieved through the use of a master/slave architecture.
I2C allows for multiple devices to be connected on the same bus, which can reduce the number of wires needed in a system. If the slave deice is not able to keep up with the processing, it may also additionally halt the clock line to keep up with the master device. In most common applications, the sensors and actuators send data to the microcontrollers using the I2C protocol. The reliability and simplicity of this protocol allows its adaptation and as an industry standard.
Application
To read the temperature sensor for the reading, the microcontroller send a signal followed by address of the sensor.
The temperature sensor would then respond with its own address, indicating that it is ready to receive commands.
The microcontroller would then send a command to read the temperature data, and the temperature sensor would respond with the requested data. This data could then be used by the microcontroller to make any associated decisions .
In this way, the I2C protocol allows to communicate with various components, including sensors and actuators, using a standardized and simple communication protocol which are widely used in following domains.
Consumer Electronics: Smartphones, tablets, and laptops use it to connect to various sensors, touch controllers, and displays to the main processor. For example, the accelerometer sensor in a smartphone uses I2C to transmit data to the processor.
Internet of Things (IoT): IoT devices use I2C between the main controller and temperature and humidity sensors, motion detectors, and light sensors.
Embedded Systems: To transmit data from the engine control unit to various sensors such as temperature and pressure sensors.
Industrial Control Systems: PID control, Robotic arms,
Medical Devices: Blood monitors, ECG machine and pulse oximeters use it for communication between sensors and the main controller.
Arduino Example
Wire library is used in Arduino to initiate the i2c protocol.
Wire.begin() function initializes the I2C interface.
To send a command to the I2C device, use the Wire.beginTransmission() function.
The first argument should be the address of the device you want to communicate with (this can be found in the device’s datasheet). The second argument should be the command you want to send to the device.
To receive data from the I2C device, use the Wire.requestFrom() function.
The first argument should be the address of the device you want to communicate with, and the second argument should be the number of bytes you expect to receive. Once you’ve called this function, you can use the Wire.read() function to read the received data.
Here’s an example code to read data from an I2C device:
#include <Wire.h>
void setup() {
Wire.begin(); // Initialize I2C interface
Serial.begin(9600); // Initialize serial communication
}
void loop() {
Wire.beginTransmission(0x__); // Start communication with the device with address 0x__
Wire.write(0x>>); // Send command to request data
Wire.endTransmission();
Wire.requestFrom(0x__, a); // Request a bytes of data from the device
if (Wire.available() == 2) {
int highByte = Wire.read(); // Read high byte of data
int lowByte = Wire.read(); // Read low byte of data
int sensordata = (highByte << 8) | lowByte; // Combine high and low bytes
Serial.print("Sensor Data ");
Serial.print(sensordata);
Serial.println(" ");
}
delay(500);
}